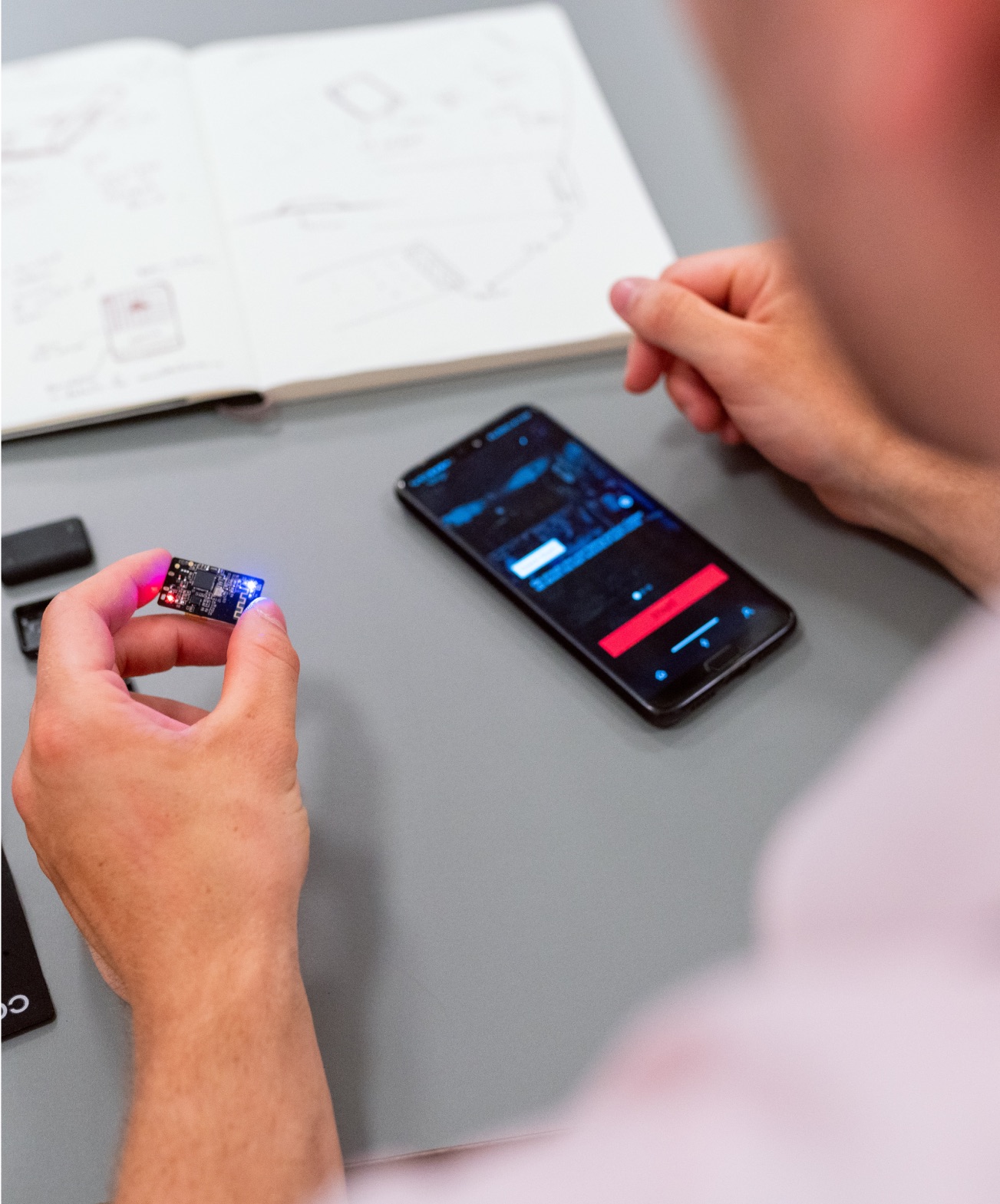
The Course
Dive into the world of modern web development with our dynamic program designed to take you from the basics of PHP and Laravel to advanced concepts that will empower you to build robust, elegant web applications. This course kicks off with the essentials, guiding you through setting up your development environment, understanding MVC architecture, and crafting your very first views and controllers. Before you know it, you'll be confidently working with databases using Eloquent ORM, handling user authentication like a pro, and learning all the ninja tricks of Blade templating.
But we won't stop at just coding - you'll learn to bring projects to life by integrating powerful features like automated testing, API development, and package management. Discover how to deploy your applications, ensuring they are scalable and secure, ready for the real world. As you journey from beginner to master, you'll gain the skills to not only craft stellar applications but also to stand out in a competitive job market. Let's turn that curiosity into capability and get ready to conquer the digital landscape with your newfound Laravel prowess!
What you will learn
When crafting this journey for you, I meticulously curated every lesson to ensure a smooth and engaging learning experience, starting from the very basics and gradually working up to the more advanced features and practices of Laravel. I understand that as a newcomer, diving into the world of web development can be overwhelming. That's precisely why this course breaks down each concept into digestible segments, giving you a solid foundation to build upon. The modules are strategically structured to provide you with hands-on opportunities to apply what you've learned through comprehensive exercises and real-world projects. With careful guidance, you'll gain confidence in using Laravel to create robust and sophisticated web applications, ensuring that by the end of our time together, you'll not only grasp the essentials but will also be capable of exploring the more complex aspects of the framework with ease. Plus, there's plenty of additional resources and support to keep you on track, making sure that help is always at hand whenever you might need it.
Curriculum
- Creating a basic route (7:51)
- Syntax examples of routes (4:12)
- Dependency injection and redirects (5:14)
- View method and artisan route list (4:59)
- Route Parameters (3:49)
- Optional route parameters (4:25)
- Route regulars expressions - part 1 (4:49)
- Route regulars expressions - part 2 - more complex expressions (5:39)
- Route regulars expressions - part 3 - Multiple values (6:11)
- Route regulars expressions - part 4 - Constraint methods (6:03)
- Named routes (6:05)
- CSRF intro (5:06)
- Blade CSRF directive (4:25)
- CSRF methods (4:53)
- Using Laravel CSRF tokens with JS AJAX - part 1 - jQuery install (5:22)
- Using Laravel CSRF tokens with JS AJAX - part 2 - pulling values from meta (4:13)
- Using Laravel CSRF tokens with JS AJAX - part 3 - submitting post request easily (1:59)
- Using Laravel CSRF tokens with JS AJAX - part 4 - using jQuery AJAX object (3:51)
- Using the JS AXIOS library to submit AJAX requests the EASY way (10:53)
- Creating a controller with artisan (5:48)
- Creating a controller manually (8:05)
- Controller Middlewares (9:42)
- Creating a resource controller (7:07)
- Creating a resource Controller - part 2 - Store method (3:14)
- Creating a resource Controller - part 3 - Show method (4:09)
- Creating a resource Controller - part 4 - Edit method (2:27)
- Creating a resource Controller - part 5 - Update method (7:37)
- Creating a resource Controller - part 6 - Destroy method (3:29)
- Organizing multiple resource controllers (3:10)
- Partial resource routes (4:21)
- API resource routes (2:39)
- Changing resource route names (4:01)
- Naming resource routes parameters (3:21)
- Adjusting Resource URI's to your language of choice (8:51)
- Supplementing resources with additional methods (2:24)
- Getting all input data (4:51)
- Using the collect method (5:13)
- Using the input method (10:52)
- Placing default values on data returned (1:38)
- Retrieving dates as objects and using dynamic properties (10:30)
- The only and except methods (9:12)
- Checking data with the has method (3:49)
- Checking for input data missing keys (4:41)
- Supplementing inputs with more data (3:33)
- Escaping for JS (3:41)
- Blade fundamentals (5:04)
- If stattements (5:02)
- Switch statements (1:45)
- Loops (7:05)
- Continue and Break (6:55)
- Loops variable (5:25)
- Conditional classes (4:35)
- Conditional styles (1:33)
- The Blade include directive (2:49)
- Including with conditions (6:58)
- Combining loops and variables iteration in one line with each (3:06)
- Let's create a Blade master layout (7:23)
- Anonymous Component (7:44)
- Class Based Component (7:21)
- Passing data to class based Components (7:38)
- Passing data to anonymous Components part 1 (4:13)
- Passing data to anonymous Components part 2 - Dynamic data (2:44)
- Passing data to anonymous Components part 3 - Props (5:57)
- Accessing parent variables (3:19)
- Let's create a class for an Anonymous Component part 1 (7:18)
- Let's create a class for an Anonymous Component part 2 (3:15)
- Let's create a class for an Anonymous Component part 3 (5:03)
- The attributes variable (6:13)
- One more example of the attributes variable (5:52)
- Component Methods (5:03)
- Modal mini project part 1 - CSS (7:06)
- Modal mini project part 2 - Routes and CSS styling (6:36)
- Modal mini project part 3 - Slots (5:54)
- Modal mini project part 4 - Color property (3:44)
- Viewing component data from within the class itself (2:35)
- Modal mini project part 5 - Let's finish (16:39)
- Inline Component Views for small components (2:54)
- Dynamic component rendering (9:25)
- Intro (5:28)
- Adding and retrieving sessions (3:54)
- Creating a web route, controller and index method for sessions (4:16)
- Let's create some methods so we can play with sessions (5:12)
- Controller methods for setting values on sessions (2:23)
- More methods for retrieving and setting data into sessions (3:35)
- Storing arrays in sessions and pushing values into them (2:41)
- Deleting from arrays in sessions (9:01)
- Deleting session data by creating a collection (8:33)
- Creating a form (9:13)
- Creating a web route and resource Controller (4:20)
- Introducing the errors array (7:00)
- Error directive and CSS classes (7:39)
- Completing the body input with validation (3:38)
- Repopulating the form with flashed input data (3:24)
- Validation Exception and JSON responses (7:06)
- Form requests (4:26)
- Customizing form request rules messages (3:37)
- Creating validation manually with the Validator Class (6:21)
- Customizing rules messages with the Validator Class (5:18)
- Connecting to a SQLITE Database (5:29)
- Connecting to a MariaDB/MYSQL Database (6:11)
- PHPStorm - Data source for SQLITE databases (3:41)
- PHPStorm - Data source for MariaDB/MySQL databases (3:36)
- VS CODE - Installing DB plugin for SQLITE (2:43)
- VS CODE - install extension for MySQL/MariaDB databases (3:59)
- CRUD - inserting data using SQL queries (7:19)
- CRUD - reading data using SQL queries (2:40)
- CRUD - updating data using SQL queries (6:06)
- CRUD - Deleting data using SQL queries (2:18)
- SQL commands for the DB CLI (6:41)
- Migrate commands (6:14)
- SQlite foreign key options (2:32)
- Displaying users from - part 2 (10:40)
- Creating layouts - part 1 - styling framework and users view (8:38)
- Displaying individual user - part 3 (7:26)
- Updating our user - part 4 - starting validation form (13:35)
- Updating our user - part 5 - finishing validation form (7:21)
- Updating our user - part 6 - name and email validation rules (7:20)
- Updating our user - part 7 - advanced password validation rules (13:37)
- Updating our user - part 8 - Regular expressions (6:06)
- Updating our user - part 8 - Finally updating and time fix (17:43)
- Creating a user part 1 - forms and method (12:17)
- Creating our user - part 2 - creating the user (3:13)
- Creating our user - part 3 - adding additional data to request (9:23)
- Adding a navigation to our project (5:41)
- Finishing navigation and adding a footer (7:54)
- Deleting a user (7:58)
- Creating a custom method to add many users from a JSON file (9:37)
- Deleting and truncating custom methods (12:43)
- Using advanced select DB queries (8:02)
- Advanced fake data creation (11:00)
- Learning about offset and cursor Pagination (14:34)
- Learning to customize pagination links part 1 (7:16)
- Learning to customize pagination links part 1 - end (2:19)
- Creating a new table and migration execution (6:22)
- Intro (5:33)
- Adding a column and migrating (6:36)
- Adding a column with another migration (13:34)
- Dropping columns (8:03)
- Column ordering (6:22)
- Stepping and batching (7:05)
- Column existence and dropping logic (12:32)
- Connecting to other databases and creating tables (7:54)
- Connecting to other databases and updating tables (8:34)
- Connecting to other databases and renaming tables (1:38)
- Modifying Columns after creation (9:05)
- Renaming columns (4:50)
- Creating indexes (18:00)
- Seeding part 1 - users (6:25)
- Seeding part 2 - creating (7:23)
- Seeding part 3 - factories (9:23)
- 353-23-2-2-EMAIL-sending-email-MAILTRAP-with-styles (5:02)
- 352-23-2-1-EMAIL-sending-email-login (7:14)
- 354-23-2-3-EMAIL-sending-email-MAILTRAP-mailable (5:38)
- 355-23-2-4-EMAIL-sending-email-MAILTRAP-mailable-data (4:46)
- 356-23-2-5-EMAIL-sending-emails--using-instance (6:39)
- 357-23-2-6-EMAIL-sending-attachments (6:02)
- Authentication part 1 - setting it up (6:17)
- Database migrations (8:33)
- Authentication part 2 - everything that was created (4:09)
- Authentication part 3 - creating auth routes (4:54)
- Creating form for validation part 1 (8:47)
- Creating form for validation part 2 (6:59)
- Creating tasks (10:50)
- Displaying data part 1 (8:38)
- Displaying data part 2 (9:04)
- Deleting data (6:34)
- Deleting with policies (12:40)
- Let's integrate components part 1 (7:12)
- Let's integrate components part 2 - delete button (6:02)
- Custom user registration - (Important) (9:18)
- Accessors - two ways (6:47)
- Mutators - two ways (7:13)
- Intro and resources download (2:56)
- Laravel install and auth (4:13)
- Blade yielding and routes (8:22)
- JS and CSS imports (7:22)
- Including Sidebar in partials (10:18)
- Let's use datatable part 1 (8:21)
- Let's use datatable part 2 - Setting up data for it (7:10)
- Let's use datatable part 3 - Displaying some data (4:49)
- Let's use datatable part 4 - Displaying thousands of records (SLOW) (5:01)
- Let's use datatable part 5 - Displaying thousands of records (FAST) (7:51)
- Eager loading full explanation (7:02)
- Laravel Redirect Issue addressed (5:23)
- Home Layout (4:27)
- Linking CSS (10:58)
- Linking JS and displaying posts part 1 (5:59)
- Displaying posts part 2 (4:01)
- Pagination (9:20)
- Individual post (8:17)
- Route model binding (3:56)
- Creating more views and nav links (7:56)
- Custom logout (10:03)
- Masthead feature part 1 - Service providers (6:37)
- Masthead feature part 2 - View composers (6:23)
- Masthead feature part 3 - Done (4:55)
- Pretty URL's (Slug) part 1 (5:39)
- Pretty URL's (Slug) part 2 (6:03)
- Controller for home route (4:30)
- Creating a nav partial and session lifetime settings (8:57)
- Login in manually (10:49)
- Setting up email sending for password reset (4:36)
- Login form part 1 (11:25)
- Login form part 2 - custom routes (9:22)
- Login form part 3 - validation (5:39)
- Custom redirect in the middleware (FIX) (1:34)
- Password recovery form part 1 (7:37)
- Password recovery form part 2 (5:42)
- Sending email link (11:03)
- Password reset part 1 (9:00)
- Password reset part 2 - override email link (8:39)
- Password reset part 3 - validation (6:45)
- Password reset part 4 - updating password logic (6:59)
- Password reset part 5 - reseting - everything working (8:18)
- Custom registration part 1 (8:37)
- Custom registration part 2 (6:20)
- Custom registration part 3 - validation (4:34)
- Custom registration part 4 - dynamic property creation (20:55)
- Custom username (8:22)
- Updating route links for the forms (3:04)
- Redirecting when authenticated (6:19)
- Intro (14:07)
- What we are building (2:22)
- Alpine (8:30)
- Livewire (14:14)
- Seeding and migration (7:24)
- Displaying posts part 1 - creating posts component (7:54)
- Displaying posts part 2 - creating the html (7:01)
- Displaying posts part 3 - continued work on the html (5:00)
- Displaying posts part 3 - displaying posts from DB (5:04)
- Creating a delete button (6:16)
- Deleting a post with livewire (6:34)
- Working on the create form part 1 (6:39)
- Working on the create form part 2 (9:36)
- Tailwind CSS working on page columns (11:39)
- Cleaning up (4:11)
- Validation and pagination (7:13)
- Livewire events (15:47)
- Deleting confirmations and policies (8:59)
- Updating - edit property and toggle part 1 (7:16)
- Updating - form input styles (9:29)
- Updating - binding input values (5:31)
- Updating complete (6:34)
- Different ways to delete a post (5:45)
- Livewire forms - create method part 1 (7:38)
- Livewire forms - create method part 2 (4:42)
- Livewire forms - updating (14:31)
- Livewire and Bootstrap intro (3:28)
- Using Livewire and Bootstrap (7:48)
- What we are building in this section (1:10)
- Creating back-end and front-end (7:31)
- Installing tailwindcss (14:12)
- Creating a HTML table for displaying posts part 1 (9:11)
- Creating a HTML table for displaying posts part 2 (5:15)
- Seeding and migration (11:23)
- Creating a Post resource and transforming data (11:43)
- Displaying posts part 1 (8:58)
- Displaying posts part 2 (8:26)
- Setting up Vue.js routing part 1 (8:13)
- Setting up Vue.js routing part 2 (4:28)
- Composables (6:35)
- Deleting part 1 (6:51)
- Deleting part 2 (8:47)
- Creating posts - styling button (9:25)
- Creating posts - styling form (9:13)
- Setting up form to submit data (9:40)
- Creating a try and catch and route for submitting posts (2:39)
- Applying a CORS helper Middleware (7:06)
- Redirection and latest first (8:29)
- Fixing some middleware issues (13:07)
- Creating a post (6:11)
- Updating part 1 - routes and controller method (5:44)
- Updating part 2 - front-end link and router path (4:40)
- Updating part 3 (7:49)
- Updating part 4 (7:29)
- Updating part 5 - Finished (5:59)
- Refactoring (2:52)
Your instructor
At Coding Faculty, we pride ourselves on delivering top-notch educational resources, diligently crafted to empower students in navigating the exciting realm of web development. Since our inception in 2015, under the visionary guidance of entrepreneur, esteemed instructor, and avid blogger, Edwin Diaz, we've curated a vibrant community of expert educators committed to imparting the most comprehensive and impactful training available online.
My personal journey in web development, enriched with years of hands-on experience and a fervent love for teaching, equips me uniquely to lead our flagship "New Laravel for Beginners to Mastery Bootcamp." Each lesson is infused with my passion for the subject and my dedication to helping learners forge a deep, practical connection with the material, ensuring that the transition from novice to adept is not just educational, but truly transformative.

Comprehensive
From Novice to Expert: The Ultimate Journey with Laravel
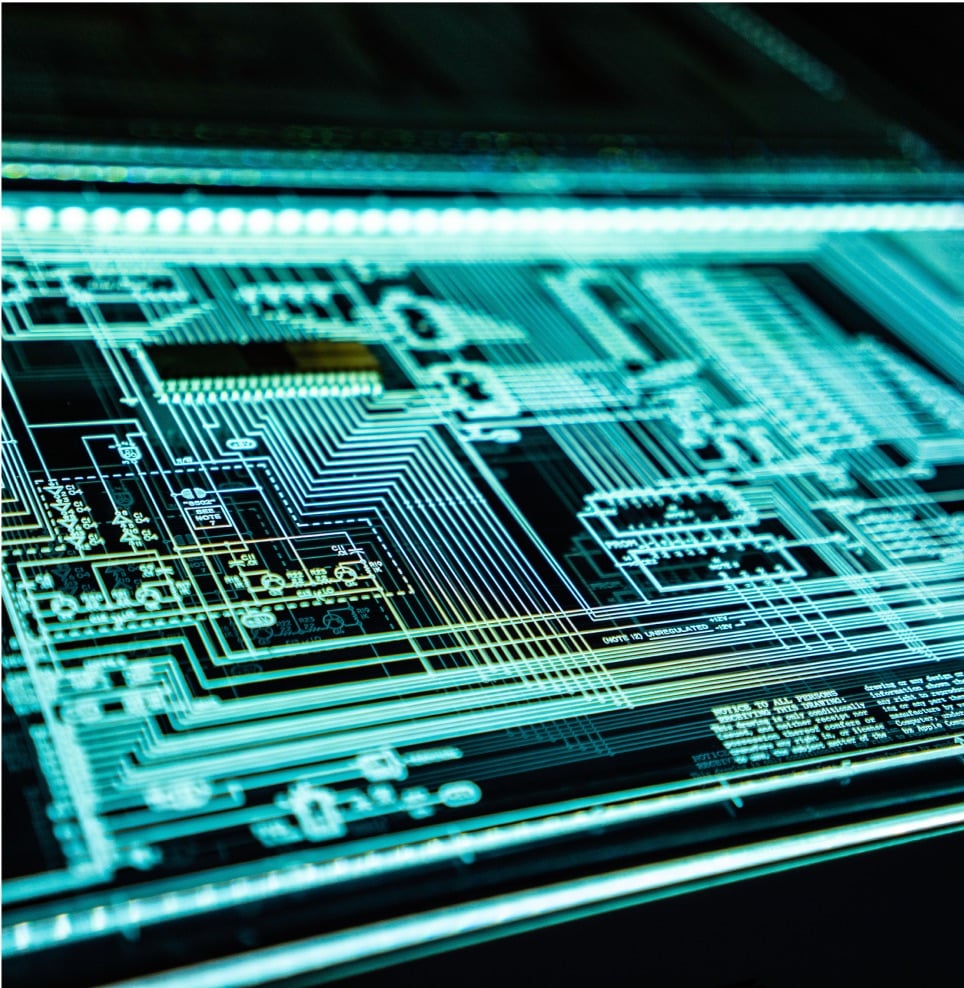
Innovative
Unleashing the New Features of Laravel for Progressive Web Development
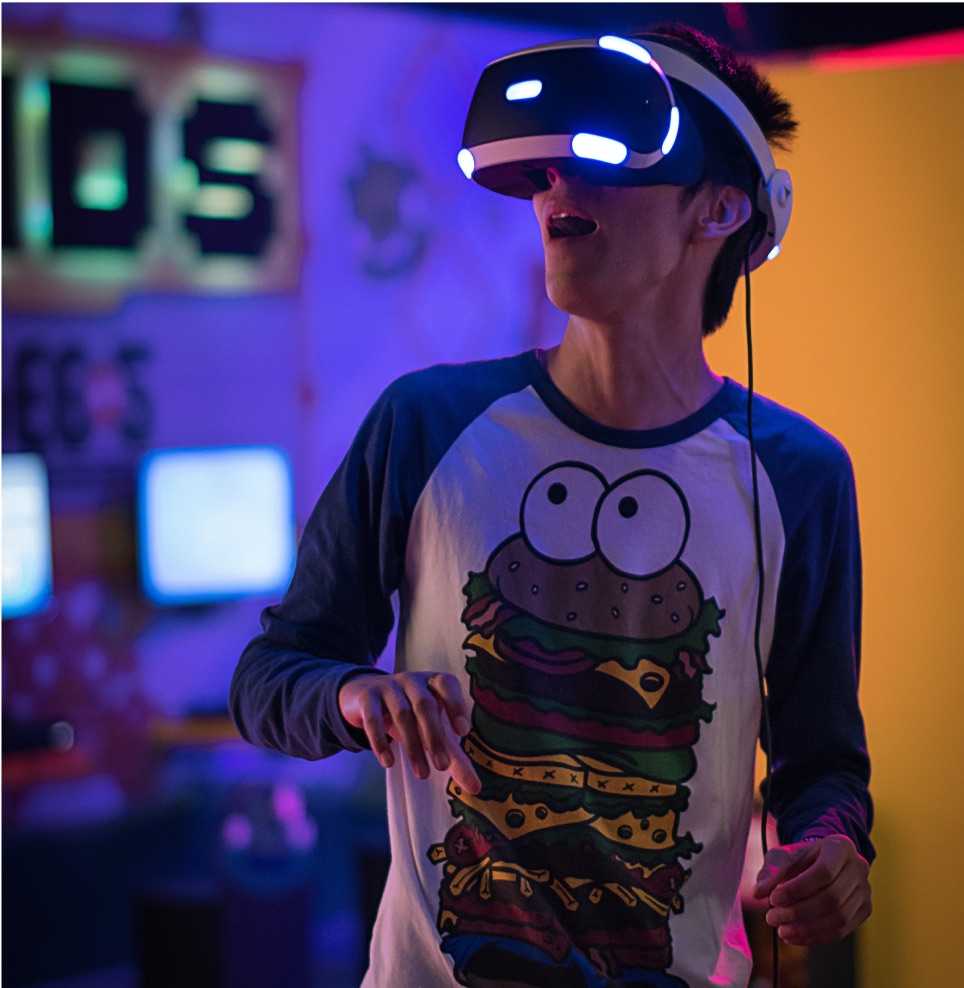
Intuitive
Mastering Laravel with Ease: A Beginner-Friendly Approach to Modern Web Apps